Our experienced Java Bootcamp London trainers are award winners!
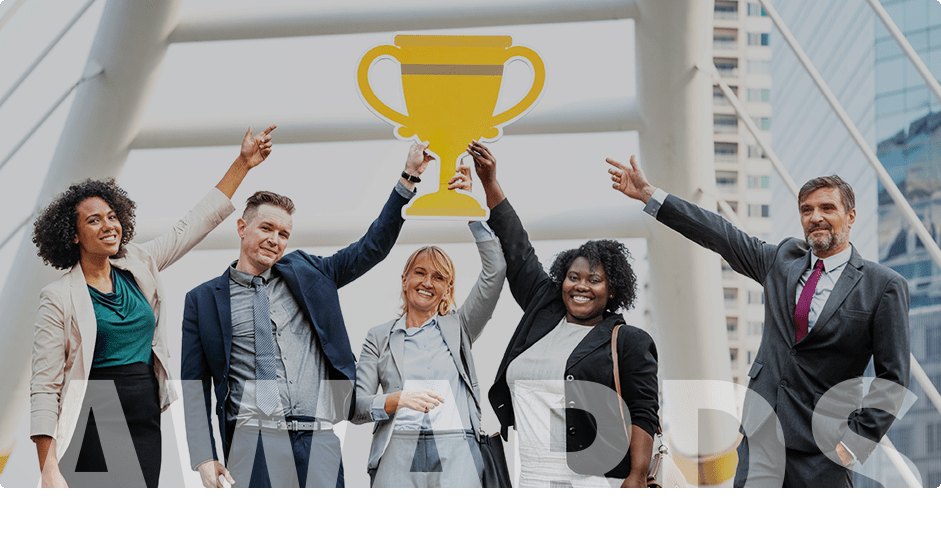
About the award
More about Java Bootcamp London
FAQ's
Client Comments
Highlights of the Java Bootcamp?
Part Time.You can work at the same time, it is a part time course with only one 1-day lesson per week. You need to have studey time between sessions.
Personalised.You will have a mentor that will work 1-1 between sessions, to make sure that you stay in line with the course and gain the skills, and answer you Java-related questions.
Practical .There is a practical Java project to give you full confidence that you have achieved a practical level of knowledge.
Much Fun! .We aim to give you a very enjoyable and useful experience and look forward to building with you Java career progress.
Processes and Threads, Thread Objects
Defining and Starting a Java Thread, Pausing Execution with Sleep
Interrupts, Joins
Synchronization, Thread Interference, Memory Consistency Errors, Synchronized Methods
Intrinsic Locks and Synchronization,
Atomic Access, Liveness, Deadlock, Starvation and Livelock
Executors, Executor Interfaces, Thread Pools, Fork/Join, Concurrent Collections
Guarded Blocks, Immutable Objects